Setup - Polling
Set up your environment to utilise Immutable's Blockchain Data to get data on assets, collections, tokens and more.
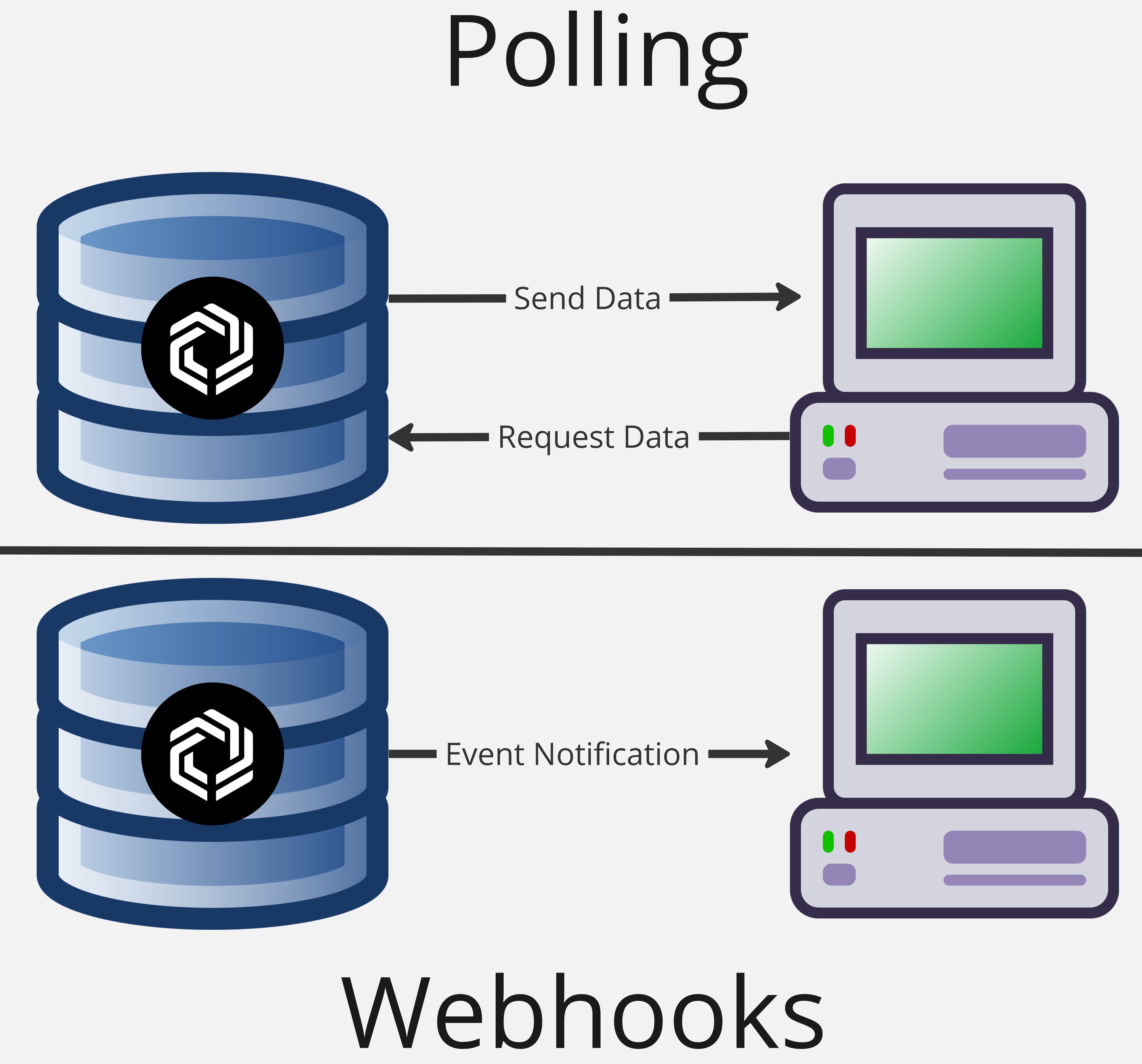
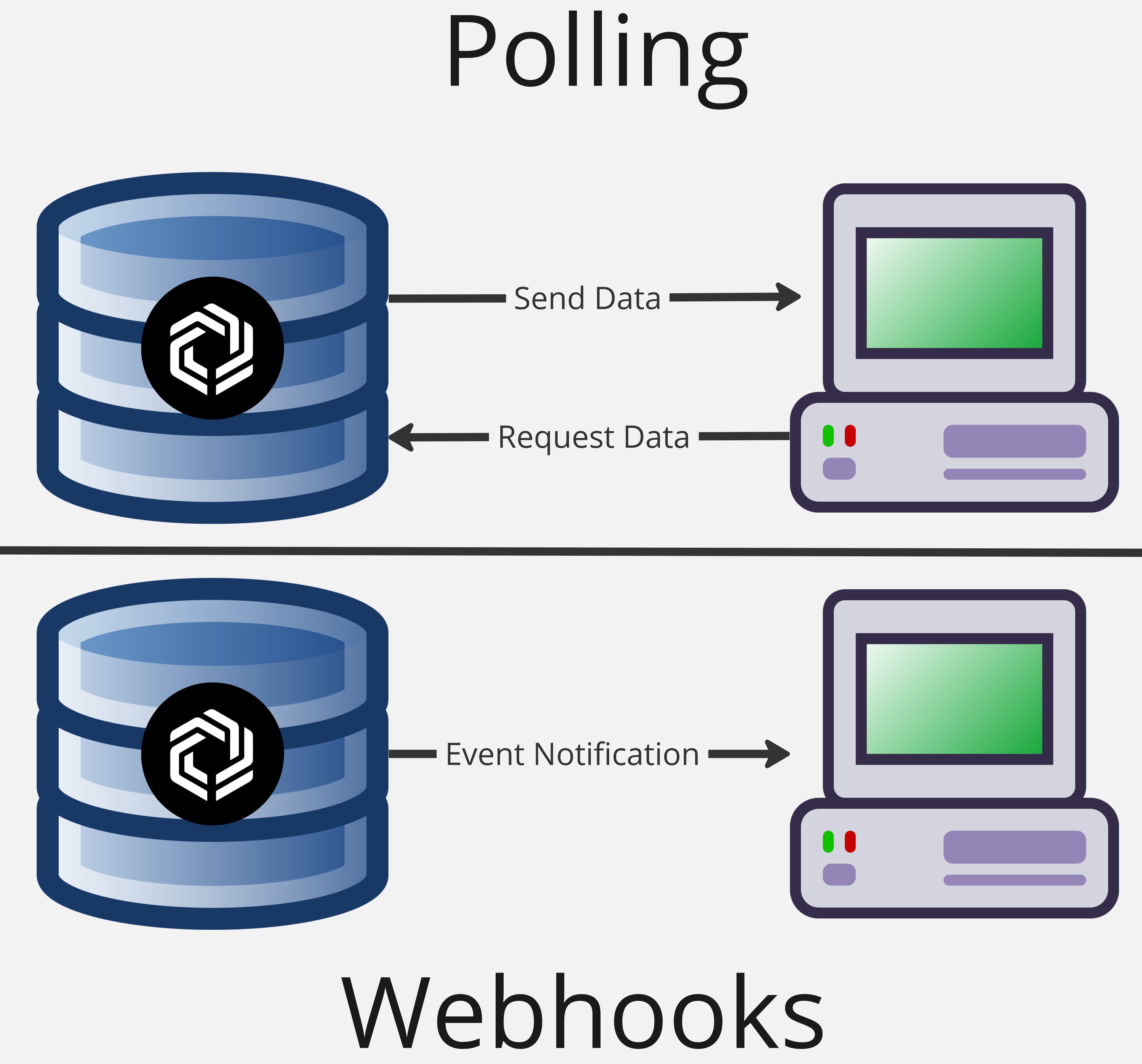
Create project and install and initialize Immutable SDK
The following guides are for how to use the Immutable SDK in a JavaScript project. Alternatively, you can use the Immutable API.
1. Create a project (or use an existing one)
# Create a folder for your project
mkdir myproject && cd myproject
# Initalise a js project
# Use the -y flag to accept the default setup
npm init -y
2. Install dependencies
Prerequisites
Node Version 20 or later
To install nvm
follow these instructions. Once installed, run:
nvm install --lts
- (Optional) To enable Code Splitting (importing only the SDK modules you need) there are additional prerequisites.
Install the Immutable SDK
Run the following command in your project root directory.
- npm
- yarn
npm install -D @imtbl/sdk
# if necessary, install dependencies
npm install -D typescript ts-node
yarn add --dev @imtbl/sdk
# if necessary, install dependencies
yarn add --dev typescript ts-node
The Immutable SDK is still in early development. If experiencing complications, use the following commands to ensure the most recent release of the SDK is correctly installed:
- npm
- yarn
rm -Rf node_modules
npm cache clean --force
npm i
rm -Rf node_modules
yarn cache clean
yarn install
3. Initialize
Create an instance of the BlockchainData
module in order to start using
- Typescript
import { config, blockchainData } from '@imtbl/sdk';
const PUBLISHABLE_KEY = 'YOUR_PUBLISHABLE_KEY'; // Replace with your Publishable Key from the Immutable Hub
const client = new blockchainData.BlockchainData({
baseConfig: {
environment: config.Environment.SANDBOX,
publishableKey: PUBLISHABLE_KEY,
},
});
Core concepts

Updating blockchain data
The Blockchain Data Indexer caches both on- and off-chain asset data, providing applications with the ability to query it in a standardised format. The Indexer can receive updates about the following:
- Collection deployment: When an NFT collection is deployed, the Indexer is automatically notified to retrieve both its on and off-chain data.
- Token minted: When a new token is minted, the Indexer is automatically notified to retrieve the existing off-chain metadata for its corresponding collection and save it for the newly minted token.
- Metadata refresh: In cases where metadata (on and off-chain) is updated for NFTs or collections, the Indexer must be manually triggered to perform a metadata refresh. Please note that this feature is not currently available but it is coming soon.
For all data updates performed by the Indexer, there will be a slight delay between the event or trigger and the Blockchain Data APIs reflecting the updated data. Correspondingly, if an update is made and a manual trigger is required but was not initiated, data returned from the Blockchain Data API queries will not reflect the updated data.
Pagination
Paginated functions return their result in the following format:
{
"page": {
"previous_cursor": PREVIOUS_CURSOR,
"next_cursor": NEXT_CURSOR
},
"result": [
...data
]
}
The values returned in previous_cursor
and next_cursor
can be used as query parameter values to obtain the previous
or next page respectively. Inserting these parameters into the page_cursor
variable for the list functions will return
the desired page result.