Get collection data
A collection is a deployed ERC721 smart contract that is used to mint an in-game assets (i.e. NFTs). You can get a list of deployed collections on a specific chain, for a particular NFT owner account address, or the details of a specific collection.
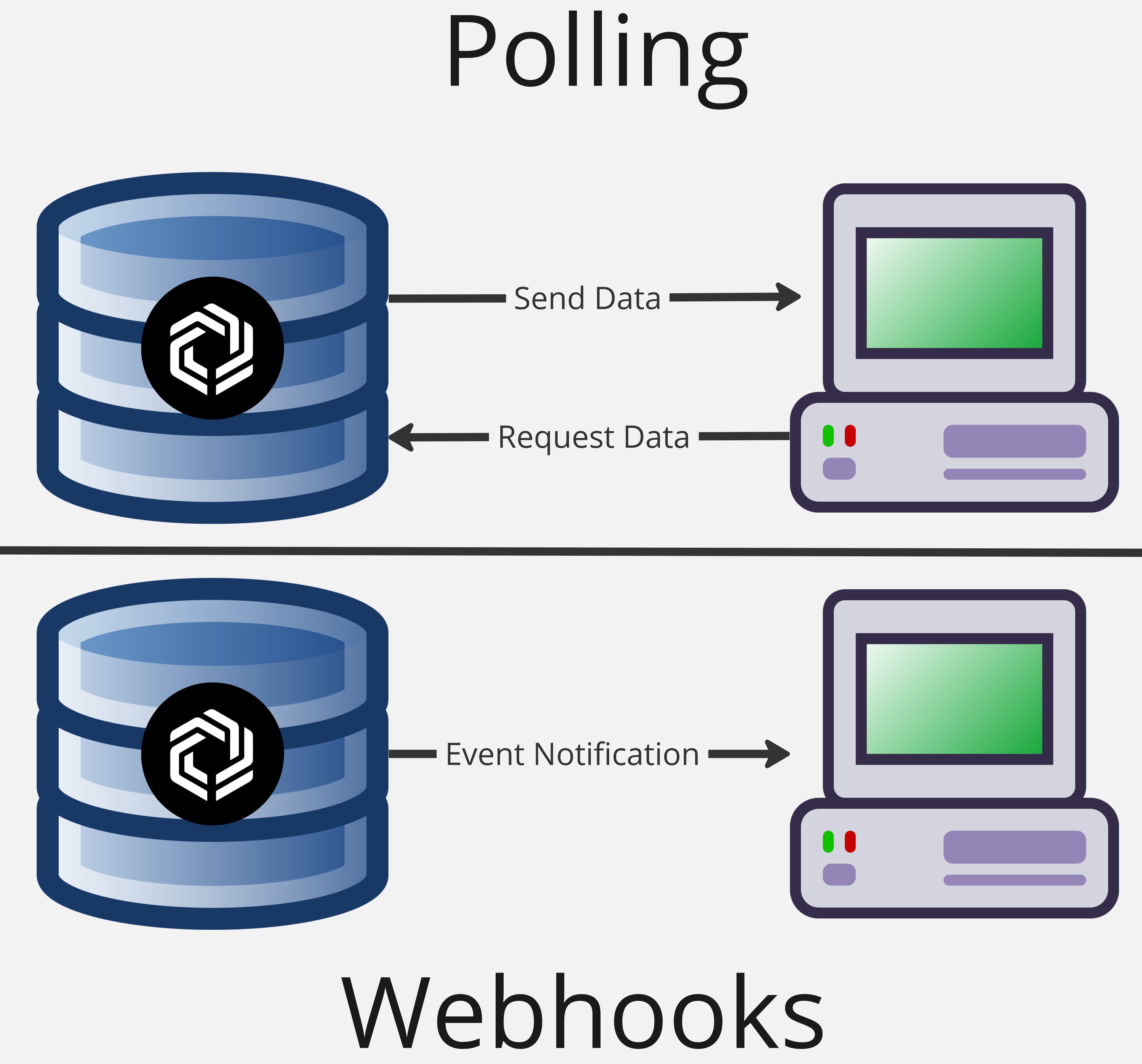
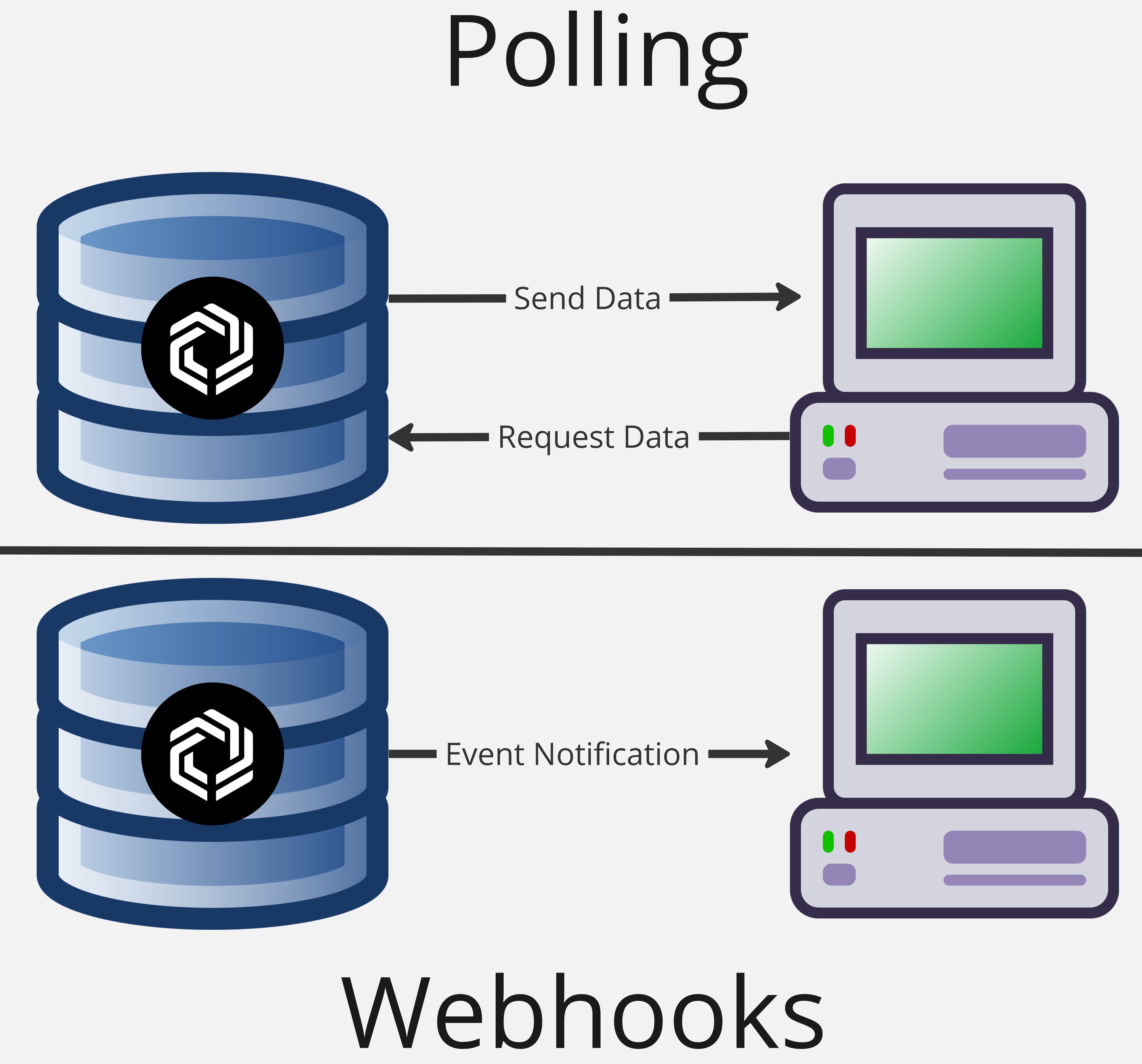
Request parameters
Parameter | Description | Required |
---|---|---|
chainName | String representing the name of the chain. A list of available chains can be found here. | Yes |
accountAddress | The public key of a wallet holding NFTs; mandatory for owner-based requests | No |
contractAddress | Optional list of contract addresses to retrieve only a subset of collections | No |
Pagination parameters | Parameters such as page_size and page_cursor that allow you to control the size and order of the data retrieved | No |
List collections on a specific chain
This request returns a list of collections deployed on a user specified chain, including on and off-chain metadata.
- SDK
- API
Use the following parameters in your function if you want to get a list of collections deployed on a specific chain:
import { blockchainData } from '@imtbl/sdk';
const listCollections = async (
client: blockchainData.BlockchainData,
chainName: string,
contractAddress: string[]
) => {
const collections = await client.listCollections({
chainName,
contractAddress,
});
};
The result will return an array of Collection objects.
Using Immutable's API you can get a list of deployed collections from an individual chain with the following ListCollections API call; which will produce a result in the below format.
GET /chains/{chainName}/collections
Example response
[
{
"result": {
"chain": {
"id": "eip155:13473",
"name": "imtbl-zkevm-testnet"
},
"name": "0x8a90cab2b38dba80c64b7734e58ee1db38b8992e",
"symbol": "BASP",
"contract_type": "ERC721",
"contract_address": "0x8a90cab2b38dba80c64b7734e58ee1db38b8992e",
"description": "Some description",
"image": "https://some-url",
"external_link": "https://some-url",
"contract_uri": "https://some-url",
"base_uri": "https://some-url",
"indexed_at": "2022-08-16T17:43:26.991388Z",
"updated_at": "2022-08-16T17:43:26.991388Z",
"last_metadata_synced_at": "2022-08-16T17:43:26.991388Z"
}
}
]
List collections by NFT owner
This request returns a list of collections deployed on a user specified chain, according to the NFT's that an account address owns.
- SDK
- API
Use the following parameters in your function if you want to get a list of collections for a particular NFT owner account address:
import { blockchainData } from '@imtbl/sdk';
const listCollectionsByNFTOwner = async (
client: blockchainData.BlockchainData,
chainName: string,
accountAddress: string,
) => {
const ownerCollections = await client.listCollectionsByNFTOwner({
chainName,
accountAddress,
});
};
The result will return an array of Collection objects.
Using Immutable's API you can get a list of collections for a particular NFT owner account address with the following listCollectionsByNFTOwner API call; which will produce a result in the below format.
GET /chains/{chainName}/accounts/{accountAddress}/collections
Example response
[
{
"result": {
"chain": {
"id": "eip155:13473",
"name": "imtbl-zkevm-testnet"
},
"name": "0x8a90cab2b38dba80c64b7734e58ee1db38b8992e",
"symbol": "BASP",
"contract_type": "ERC721",
"contract_address": "0x8a90cab2b38dba80c64b7734e58ee1db38b8992e",
"description": "Some description",
"image": "https://some-url",
"external_link": "https://some-url",
"contract_uri": "https://some-url",
"base_uri": "https://some-url",
"indexed_at": "2022-08-16T17:43:26.991388Z",
"updated_at": "2022-08-16T17:43:26.991388Z",
"last_metadata_synced_at": "2022-08-16T17:43:26.991388Z"
}
}
]
Get the details of a single collection
This request allows you to get details of a specific collection.
- SDK
- API
Use the following parameters in your function if you want to get get details of a specific collection:
import { blockchainData } from '@imtbl/sdk';
const getCollection = async (
client: blockchainData.BlockchainData,
chainName: string,
contractAddress: string
) => {
const collection = await client.getCollection({
chainName,
contractAddress,
});
};
The result will return a Collection object.
Using Immutable's API you can get the details of a specific collection with the following GetCollection API call; which will produce a result in the below format.
GET /chains/{chainName}/collections/{contractAddress}
Example response
{
"result": {
"chain": {
"id": "eip155:13473",
"name": "imtbl-zkevm-testnet"
},
"name": "0x8a90cab2b38dba80c64b7734e58ee1db38b8992e",
"symbol": "BASP",
"contract_type": "ERC721",
"contract_address": "0x8a90cab2b38dba80c64b7734e58ee1db38b8992e",
"description": "Some description",
"image": "https://some-url",
"external_link": "https://some-url",
"contract_uri": "https://some-url",
"base_uri": "https://some-url",
"indexed_at": "2022-08-16T17:43:26.991388Z",
"updated_at": "2022-08-16T17:43:26.991388Z",
"last_metadata_synced_at": "2022-08-16T17:43:26.991388Z"
}
}
When an asset is minted, its metadata is cached by Immutable's blockchain data indexer, making it accessible via the the Blockchain Data APIs.
When an NFT's metadata is updated, requesting a "metadata refresh" updates the cached information to ensure that changes are available via the API.