Get & update metadata
Immutable's Blockchain Data APIs allows developers and 3rd parties to access all collection's off-chain metadata in a centralised location. Metadata is essential in defining the context, characteristics, and aesthetics of NFTs.
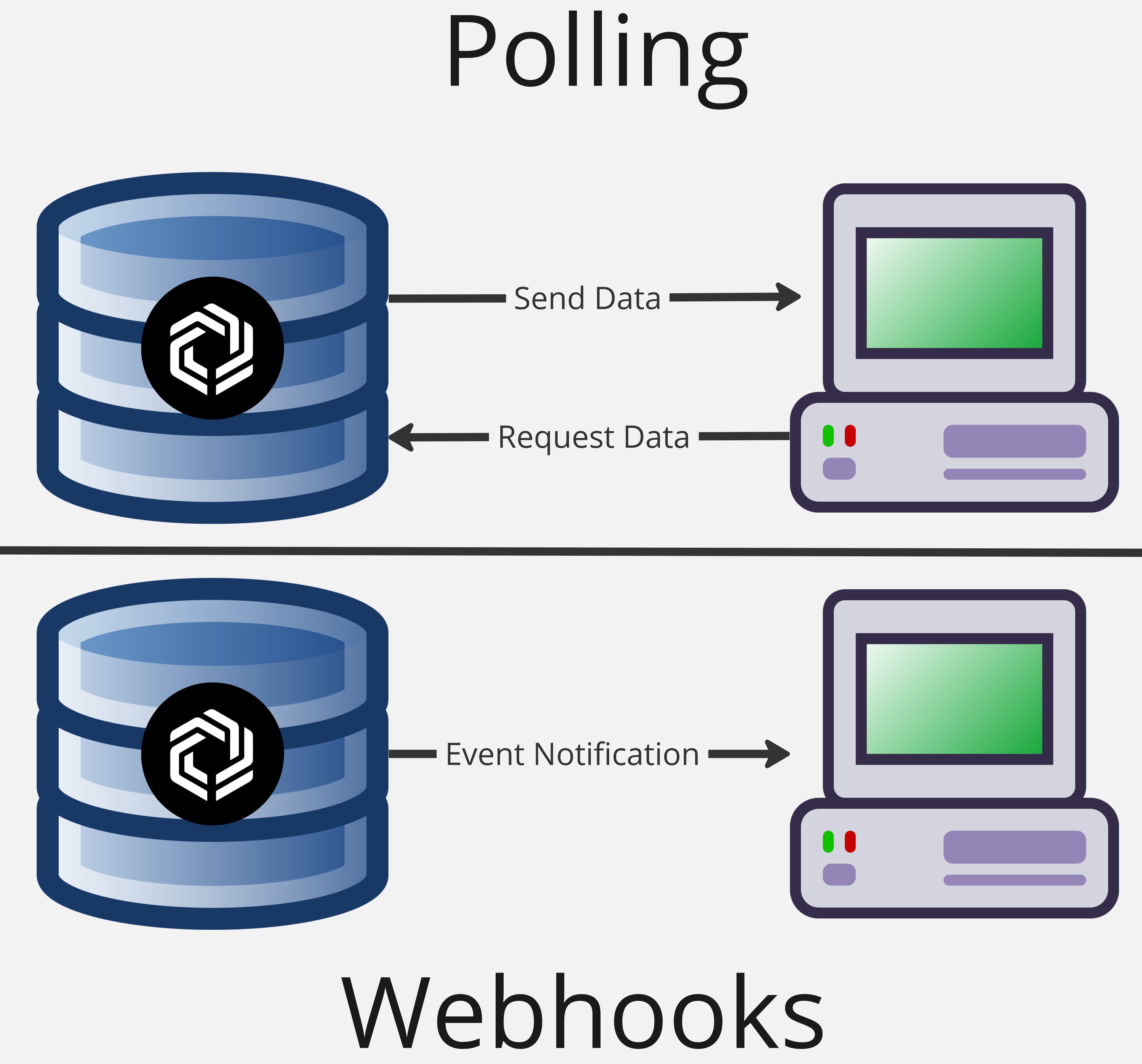
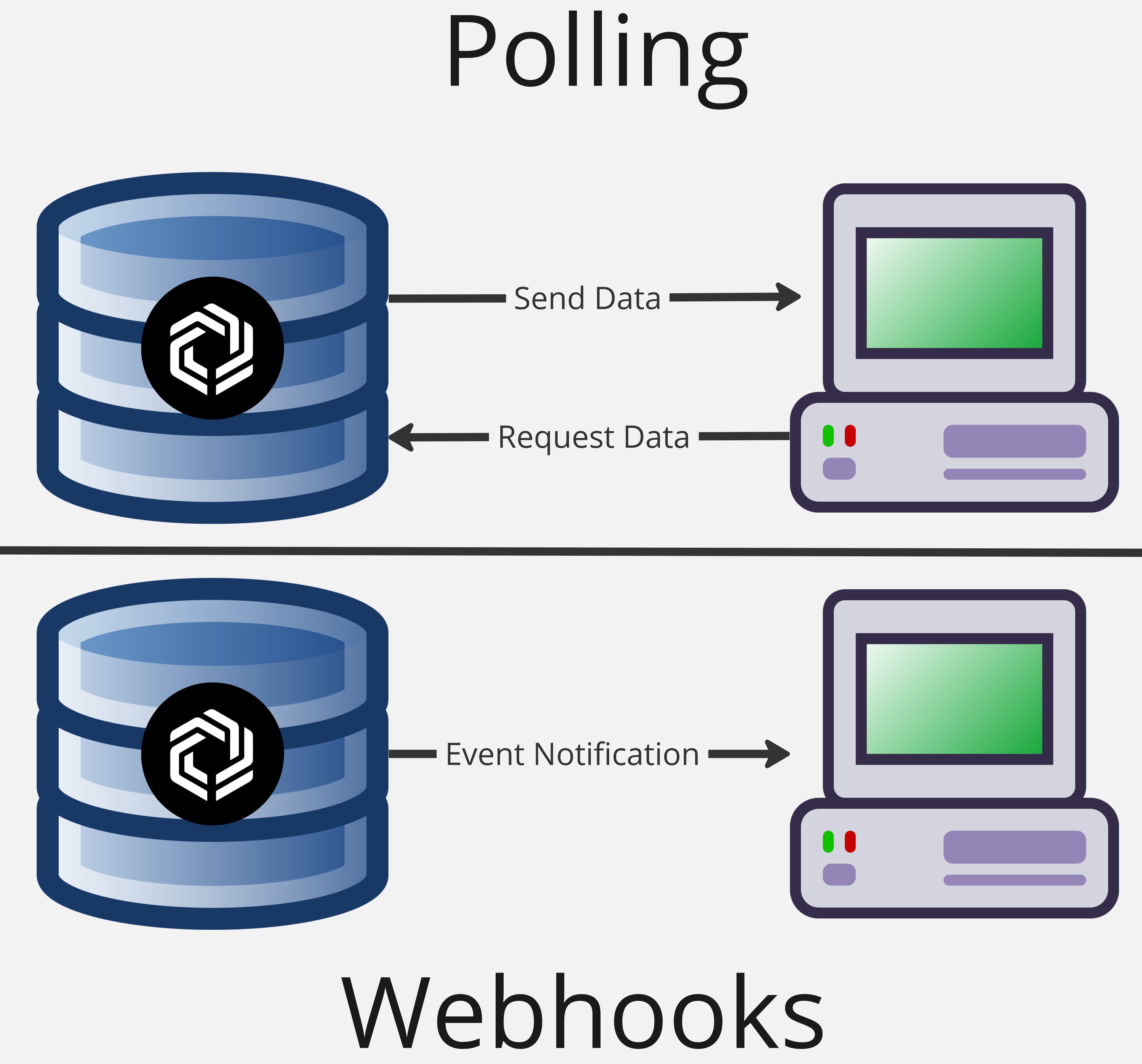
Request parameters
Parameter | Description | Required |
---|---|---|
chainName | String representing the name of the chain. A list of available chains can be found here | Yes |
contractAddress | The contract address of ERC721 contract you want to filter by; mandatory for collection-based requests, including metadata refresh requests | No |
accountAddress | The Ethereum address of a wallet holding NFTs; mandatory for owner-based requests | No |
Pagination parameters | Parameters such as page_size and page_cursor that allow you to control the size and order of the data retrieved | No |
Get a single metadata
This request allows you to get details of a specific metadata object referenced by a metadata_id. A metadata_id corresponds to the metadata of all stacked NFTs that shares the same metadata attributes.
- SDK
- API
Use the following parameters in your function if you want to get a specific metadata for a given collection:
import { blockchainData } from '@imtbl/sdk';
const getMetadata = async (client: blockchainData.BlockchainData, chainName: string, contractAddress: string, metadataId: string) => {
const metadata = await client.getMetadata({
chainName,
contractAddress,
metadataId,
});
};
The result will return a Metadata object.
Using Immutable's API you can get a specific metadata for a given collection with the following GetMetadata API call; which will produce a result in the below format.
GET /chains/{chain_name}/collections/{contract_address}/metadata/{metadata_id}
Example response
{
"result": {
"id": "4e28df8d-f65c-4c11-ba04-6a9dd47b179b",
"chain": {
"id": "eip155:13473",
"name": "imtbl-zkevm-testnet"
},
"contract_address": "0x8a90cab2b38dba80c64b7734e58ee1db38b8992e",
"created_at": "2022-08-16T17:43:26.991388Z",
"updated_at": "2022-08-16T17:43:26.991388Z",
"name": "Sword",
"description": "2022-08-16T17:43:26.991388Z",
"image": "https://some-url",
"external_url": "https://some-url",
"animation_url": "https://some-url",
"youtube_url": "https://some-url",
"attributes": [
{
"trait_type": "Aqua Power",
"value": "Happy"
}
]
}
}
List metadata of a specific collection
This request returns a list of metadata of a specific collection.
A metadata_id
can correlate to a single NFT or a stack of NFTs that share the same metadata attributes.
Results will be sorted by updated_at
, with the most recent entries first.
- SDK
- API
Use the following parameters in your function if you want to get a list of metadata of a specific collection:
import { blockchainData } from '@imtbl/sdk';
const listMetadata = async (client: blockchainData.BlockchainData, chainName: string, contractAddress: string) => {
const metadata = await client.listNFTMetadataByContractAddress({
chainName,
contractAddress,
});
};
The result will return an array of Metadata objects.
Using Immutable's API you can get a list of metadata for a specific collection with the following ListMetadata API call; which will produce a result in the below format.
GET /chains/{chain_name}/collections/{contract_address}/metadata
Example response
{
"result": [
{
"id": "4e28df8d-f65c-4c11-ba04-6a9dd47b179b",
"chain": {
"id": "eip155:13473",
"name": "imtbl-zkevm-testnet"
},
"contract_address": "0x8a90cab2b38dba80c64b7734e58ee1db38b8992e",
"created_at": "2022-08-16T17:43:26.991388Z",
"updated_at": "2022-08-16T17:43:26.991388Z",
"name": "Sword",
"description": "2022-08-16T17:43:26.991388Z",
"image": "https://some-url",
"external_url": "https://some-url",
"animation_url": "https://some-url",
"youtube_url": "https://some-url",
"attributes": [
{
"trait_type": "Aqua Power",
"value": "Happy"
}
]
}
],
"page": {
"previous_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0=",
"next_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0="
}
}