Metadata Search
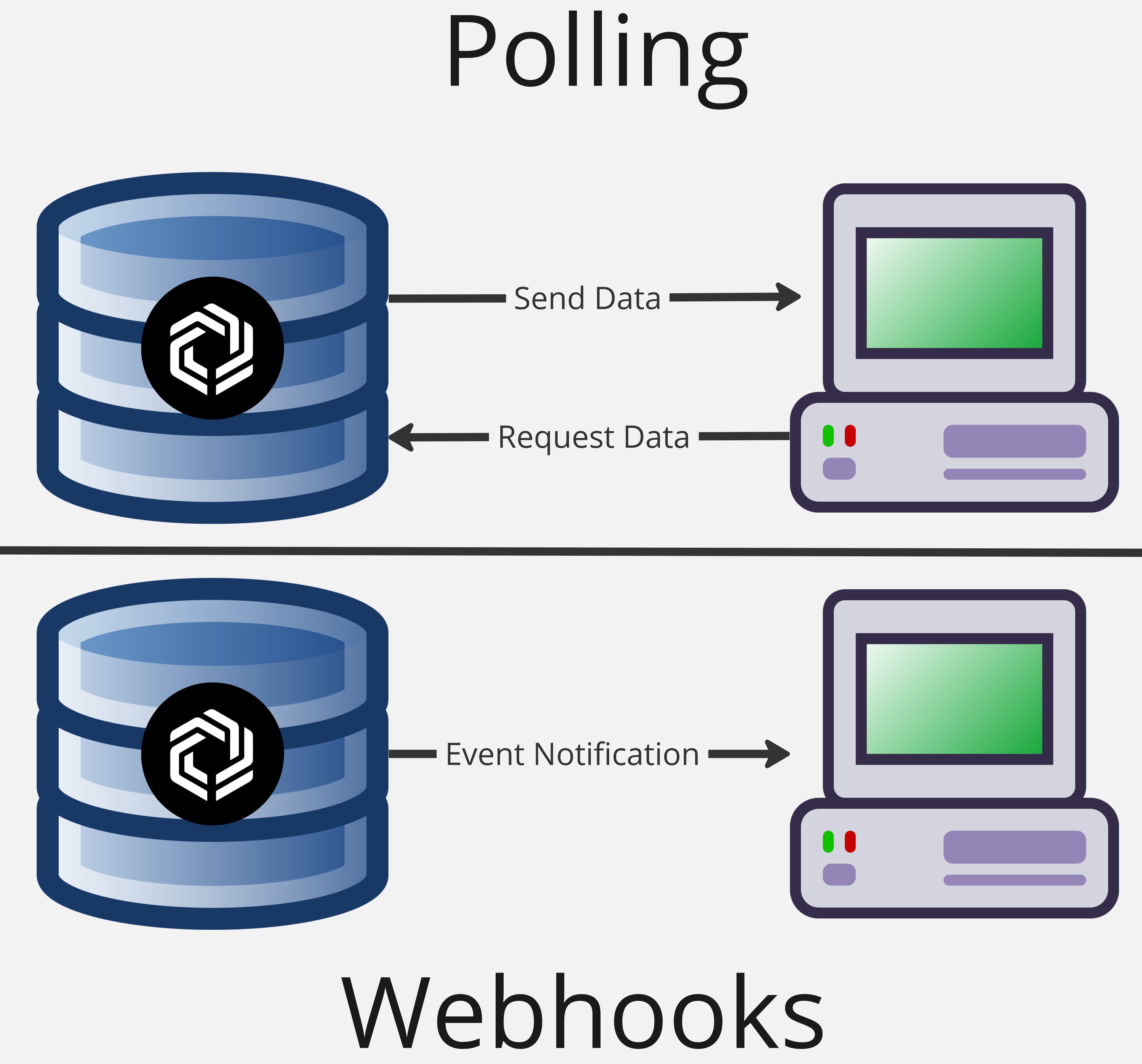
What is Metadata Search?
Metadata Search enables players to search and filter assets by metadata values as well as view market data for those assets. Typically this can be used to build a marketplace grid page as well as showing players the value of their assets in their inventory.
How does Metadata Search work?
Metadata Search is powered by our Marketplace API endpoints. See below for usage information.
Why use Metadata Search?
Metadata Search is a powerful tool for marketplaces and game studios. It boosts liquidity across the ecosystem as players can find and value desirable assets quickly. These features can be seamlessly implemented through our API endpoints which means developers only need to build the front-end of their application to be fully functional on web and in-game.
How do I implement Metadata Search?
Metadata Search utilises 3 key endpoints described below. You can leverage each one for your specific use case:
1. List metadata filters for a collection
Get the number of assets with a specific trait value grouped by metadata category filters. This gives players the ability to filter and find desired assets quickly.
Use cases
- Collection grid page to display relevant metadata filters
- Player inventory page to quickly filter, surface and group assets
Request Parameters
Parameter | Description | Required |
---|---|---|
chainName | String representing the name of the chain. A list of available chains can be found here | Yes |
contractAddress | The contract address of ERC721 contract you want to filter by | Yes |
Pagination parameters | Parameters such as page_size and page_cursor that allow you to control the size and order of the data retrieved | No |
- SDK
- API
import { blockchainData } from '@imtbl/sdk';
import { client } from '../lib';
export async function listFilters(
chainName: string,
contractAddress: string,
): Promise<blockchainData.Types.ListFiltersResult> {
return await client.listFilters({
chainName,
contractAddress,
});
}
GET /chains/{chain_name}/search/filters/{contract_address}
Example response
{
"result": {
"chain": {
"id": "eip155:13372",
"name": "imtbl-zkevm-testnet"
},
"contract_address": "0xe9b00a87700f660e46b6f5deaa1232836bcc07d3",
"filters": [
{
"name": "Rarity",
"values": [
[
{
"value": "Common",
"nft_count": "42"
},
{
"value": "Rare",
"nft_count": "17"
}
]
],
"omitted_values_count": 0
}
]
},
"page": {
"previous_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0=",
"next_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0="
}
}
2. Search for metadata and market data across collections and player inventory
Given a collection and wallet address (player inventory), get a list of metadata stacks with market listings, floor price and most recent trade details.
Use cases
- Show the price and value of assets in a collection grid page (based on last trade or floor listing)
- Show players the value of the assets they own in their inventory (based on last trade or floor listing)
- Show players the status of their current live listings
- Build auction functionality that can show all listings for a specific Metadata Stack ID
Request Parameters
Parameter | Description | Required |
---|---|---|
chainName | String representing the name of the chain. A list of available chains can be found here | Yes |
contractAddress | The contract address of ERC721 contract you want to filter by | Yes |
accountAddress | Account wallet address to filter by | No |
onlyIncludeOwnerListings | Should include only the owner created listings | No |
stackId | Metadata Stack ID to filter by | No |
Pagination parameters | Parameters such as page_size and page_cursor that allow you to control the size and order of the data retrieved | No |
- SDK
- API
import { blockchainData } from '@imtbl/sdk';
import { client } from '../lib';
export async function searchNFTs(
chainName: string,
contractAddress: string[],
): Promise<blockchainData.Types.SearchNFTsResult> {
return await client.searchNFTs({
chainName,
contractAddress,
});
}
GET /chains/{chain_name}/search/nfts
Example response
{
"result": [
{
"nft_with_stack": {
"token_id": "string",
"stack_id": "8d268dcf-caa3-4f85-9d09-6ac2a48a6fde",
"chain": {
"id": "eip155:13372",
"name": "imtbl-zkevm-testnet"
},
"contract_address": "string",
"contract_type": "ERC721",
"created_at": "2022-08-16T17:43:26.991388Z",
"updated_at": "2022-08-16T17:43:26.991388Z",
"name": "Sword",
"description": "2022-08-16T17:43:26.991388Z",
"image": "https://some-url",
"external_url": "https://some-url",
"animation_url": "https://some-url",
"youtube_url": "https://some-url",
"attributes": [
{
"display_type": "number",
"trait_type": "Aqua Power",
"value": "Happy"
}
],
"balance": 1
},
"market": {
"floor_listing": {
"listing_id": "018792C9-4AD7-8EC4-4038-9E05C598534A",
"price_details": {
"token": {
"type": "NATIVE",
"symbol": "IMX"
},
"amount": "9750000000000000000",
"fee_inclusive_amount": "9750000000000000000",
"fees": [
{
"type": "TAKER_ECOSYSTEM",
"recipient_address": "0xf39Fd6e51aad88F6F4ce6aB8827279cffFb92233",
"amount": "1000000000000000000"
}
],
"converted_prices": {
"ETH": "0.0058079775",
"USD": "15.89"
}
},
"token_id": "1",
"contract_address": "0xe9b00a87700f660e46b6f5deaa1232836bcc07d3",
"creator": "0xe9b00a87700f660e46b6f5deaa1232836bcc07d3",
"amount": "1"
},
"last_trade": {
"trade_id": "4e28df8d-f65c-4c11-ba04-6a9dd47b179b",
"contract_address": "0xe9b00a87700f660e46b6f5deaa1232836bcc07d3",
"token_id": "1",
"price_details": [
{
"token": {
"type": "NATIVE",
"symbol": "IMX"
},
"amount": "9750000000000000000",
"fee_inclusive_amount": "9750000000000000000",
"fees": [
{
"type": "TAKER_ECOSYSTEM",
"recipient_address": "0xf39Fd6e51aad88F6F4ce6aB8827279cffFb92233",
"amount": "1000000000000000000"
}
],
"converted_prices": {
"ETH": "0.0058079775",
"USD": "15.89"
}
}
],
"amount": "1",
"created_at": "2022-08-16T17:43:26.991388Z"
}
},
"listings": [
{
"listing_id": "018792C9-4AD7-8EC4-4038-9E05C598534A",
"price_details": {
"token": {
"type": "NATIVE",
"symbol": "IMX"
},
"amount": "9750000000000000000",
"fee_inclusive_amount": "9750000000000000000",
"fees": [
{
"type": "TAKER_ECOSYSTEM",
"recipient_address": "0xf39Fd6e51aad88F6F4ce6aB8827279cffFb92233",
"amount": "1000000000000000000"
}
],
"converted_prices": {
"ETH": "0.0058079775",
"USD": "15.89"
}
},
"token_id": "1",
"contract_address": "0xe9b00a87700f660e46b6f5deaa1232836bcc07d3",
"creator": "0xe9b00a87700f660e46b6f5deaa1232836bcc07d3",
"amount": "1"
}
]
}
],
"page": {
"previous_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0=",
"next_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0="
}
}
3. Search metadata and trait values
Similar to SearchNFTs
but with the ability to search and filter by metadata description, name and asset traits.
Use cases
- Show asset and pricing data based on plain text searches of asset names and descriptions
- Filter assets by metadata traits and values
- Build auction tooling to only show assets that are currently listed
Request Parameters
Parameter | Description | Required |
---|---|---|
chainName | String representing the name of the chain. A list of available chains can be found here | Yes |
contractAddress | The contract address of ERC721 contract you want to filter by | Yes |
keyword | Keyword to search NFT name and description | No |
traits | JSON encoded traits to filter by | No |
accountAddress | Account wallet address to filter by | No |
onlyIfHasActiveListings | Filters results to include only stacks that have a current active listing | No |
onlyIncludeOwnerListings | Should include only the owner created listings | No |
Pagination parameters | Parameters such as page_size and page_cursor that allow you to control the size and order of the data retrieved | No |
- SDK
- API
import { blockchainData } from '@imtbl/sdk';
import { client } from '../lib';
export async function searchStacks(
chainName: string,
contractAddress: string[],
): Promise<blockchainData.Types.SearchStacksResult> {
return await client.searchStacks({
chainName,
contractAddress,
});
}
GET /chains/{chain_name}/search/stacks
Example response
{
"result": [
{
"stack": {
"stack_id": "8d268dcf-caa3-4f85-9d09-6ac2a48a6fde",
"chain": {
"id": "eip155:13372",
"name": "imtbl-zkevm-testnet"
},
"contract_address": "string",
"contract_type": "ERC721",
"created_at": "2022-08-16T17:43:26.991388Z",
"updated_at": "2022-08-16T17:43:26.991388Z",
"name": "Sword",
"description": "2022-08-16T17:43:26.991388Z",
"image": "https://some-url",
"external_url": "https://some-url",
"animation_url": "https://some-url",
"youtube_url": "https://some-url",
"attributes": [
{
"display_type": "number",
"trait_type": "Aqua Power",
"value": "Happy"
}
]
},
"stack_count": 1,
"market": {
"floor_listing": {
"listing_id": "018792C9-4AD7-8EC4-4038-9E05C598534A",
"price_details": {
"token": {
"type": "NATIVE",
"symbol": "IMX"
},
"amount": "9750000000000000000",
"fee_inclusive_amount": "9750000000000000000",
"fees": [
{
"type": "TAKER_ECOSYSTEM",
"recipient_address": "0xf39Fd6e51aad88F6F4ce6aB8827279cffFb92233",
"amount": "1000000000000000000"
}
],
"converted_prices": {
"ETH": "0.0058079775",
"USD": "15.89"
}
},
"token_id": "1",
"contract_address": "0xe9b00a87700f660e46b6f5deaa1232836bcc07d3",
"creator": "0xe9b00a87700f660e46b6f5deaa1232836bcc07d3",
"amount": "1"
},
"last_trade": {
"trade_id": "4e28df8d-f65c-4c11-ba04-6a9dd47b179b",
"contract_address": "0xe9b00a87700f660e46b6f5deaa1232836bcc07d3",
"token_id": "1",
"price_details": [
{
"token": {
"type": "NATIVE",
"symbol": "IMX"
},
"amount": "9750000000000000000",
"fee_inclusive_amount": "9750000000000000000",
"fees": [
{
"type": "TAKER_ECOSYSTEM",
"recipient_address": "0xf39Fd6e51aad88F6F4ce6aB8827279cffFb92233",
"amount": "1000000000000000000"
}
],
"converted_prices": {
"ETH": "0.0058079775",
"USD": "15.89"
}
}
],
"amount": "1",
"created_at": "2022-08-16T17:43:26.991388Z"
}
},
"listings": [
{
"listing_id": "018792C9-4AD7-8EC4-4038-9E05C598534A",
"price_details": {
"token": {
"type": "NATIVE",
"symbol": "IMX"
},
"amount": "9750000000000000000",
"fee_inclusive_amount": "9750000000000000000",
"fees": [
{
"type": "TAKER_ECOSYSTEM",
"recipient_address": "0xf39Fd6e51aad88F6F4ce6aB8827279cffFb92233",
"amount": "1000000000000000000"
}
],
"converted_prices": {
"ETH": "0.0058079775",
"USD": "15.89"
}
},
"token_id": "1",
"contract_address": "0xe9b00a87700f660e46b6f5deaa1232836bcc07d3",
"creator": "0xe9b00a87700f660e46b6f5deaa1232836bcc07d3",
"amount": "1"
}
]
}
],
"page": {
"previous_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0=",
"next_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0="
}
}