Swap tokens
The SWAP flow simplifies the process of swapping tokens on the Immutable zkEVM network.
In this section, we'll show you how to implement this flow in your application.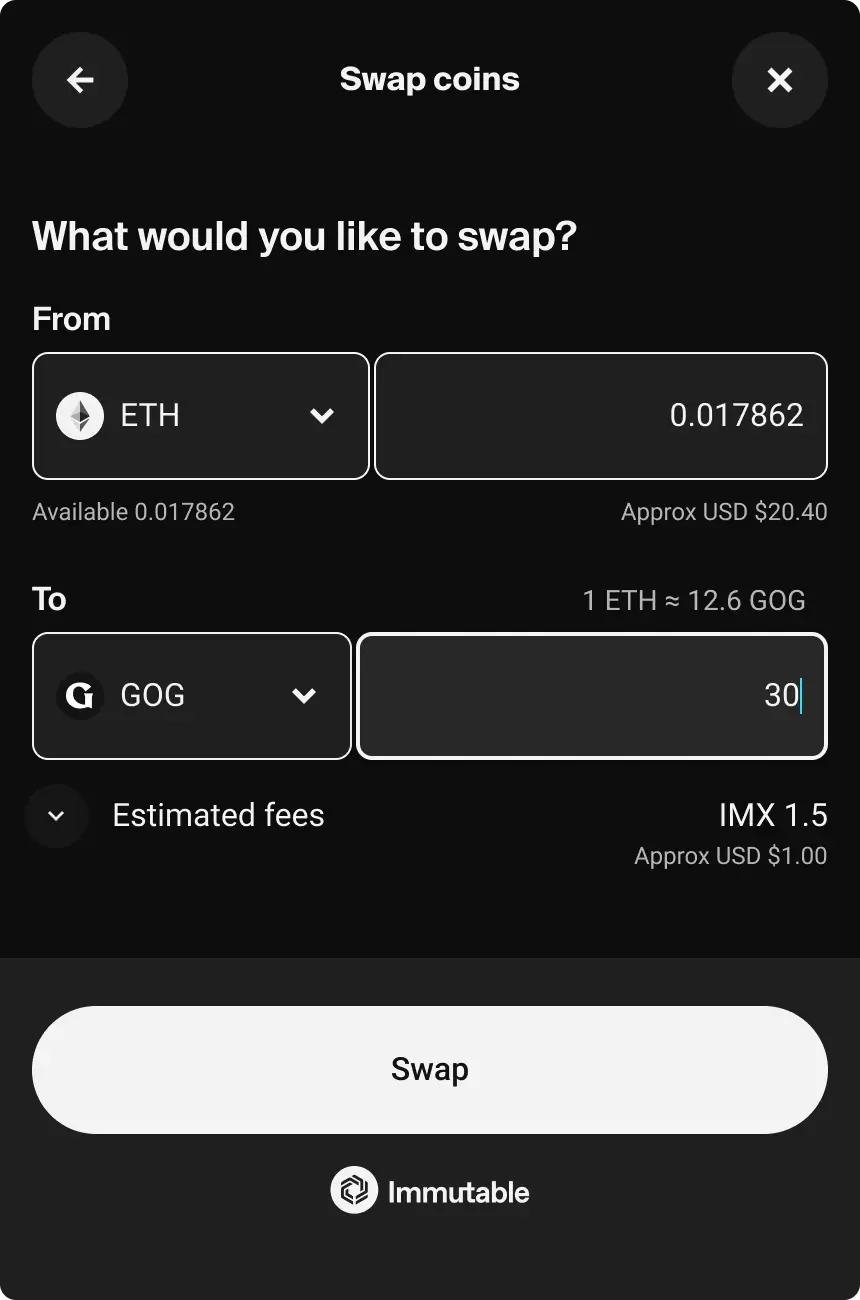
For additional questions, please contact our support team on Discord.
Getting started
Once you have completed the setup,
In order to initiate a flow call the mount()
function passing in the id
attribute of the target element you wish to mount the widget to,
and the parameters required by the SWAP
flow.
- React
- JavaScript
import { useEffect } from 'react';
import { checkout } from '@imtbl/sdk';
// Create a Checkout SDK instance
const checkoutSDK = new checkout.Checkout();
export function App() {
// Initialise the Commerce Widget
useEffect(() => {
(async () => {
// Create a factory
const factory = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK, language: 'en' },
});
// Create a widget
const widget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE);
// Mount a SWAP flow, optionally pass any SwapWidgetParams
widget.mount('mount-point', {
flow: checkout.CommerceFlowType.SWAP,
});
})();
}, []);
return <div id="mount-point" />;
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/checkout-sdk/dist/browser/index.js"></script>
</head>
<body>
<div id="mount-point"></div>
<script>
// Initialize Checkout SDK
var checkout;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const factory = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const widget = factory.create(
ImmutableCheckout.WidgetType.IMMUTABLE_COMMERCE
);
widget.mount('mount-point', {
flow: ImmutableCheckout.CommerceFlowType.SWAP,
});
})();
</script>
</body>
</html>
Parameters
The mount()
function will take in a commercewidgetswapFlowParams object.
Parameters are treated as transient and will be reset after the widget is unmounted.
Property | Type | Description |
---|---|---|
flow | CommerceFlowType.SWAP | The flow type to be used. |
amount | string | The ERC20 token amount to swap. |
fromTokenAddress | string | The address of the ERC20 contract swapping from. Use NATIVE to set the swapping from token to IMX . This will prefill the token field in the form. |
toTokenAddress | string | The address of the ERC20 contract swapping to. Use NATIVE to set the swapping to token to IMX . This will prefill the token field in the form. |
walletProviderName | WalletProviderName | The wallet provider name to use for the swap widget. |
autoProceed | boolean | Whether the swap widget should display the form or automatically proceed with the swap. |
direction | SwapDirection | The direction of the swap SwapDirection.FROM or SwapDirection.TO |
showBackButton | boolean | Whether to show a back button on the first screen, on click triggers REQUEST_GO_BACK event |
- React
- JavaScript
import { checkout } from '@imtbl/sdk';
// @ts-ignore
const widget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE, {
config: { theme: checkout.WidgetTheme.DARK },
});
// When calling the mount function,
// set flow to SWAP and pass in the parameters to use
widget.mount('mount-point', {
flow: checkout.CommerceFlowType.SWAP,
// set amounts and tokens to swap from and to
amount: '100',
direction: checkout.SwapDirection.FROM,
fromTokenAddress: 'native', // IMX
toTokenAddress: '0x3B2d8A1931736Fc321C24864BceEe981B11c3c57', // USDC
});
// When calling the mount function, pass in the parameters to use
widget.mount('mount-point', {
flow: ImmutableCheckout.CommerceFlowType.SWAP,
// set amounts and tokens to swap from and to
amount: '100',
direction: ImmutableCheckout.SwapDirection.FROM,
fromTokenAddress: 'native', // IMX
toTokenAddress: '0x3B2d8A1931736Fc321C24864BceEe981B11c3c57', // USDC
});
Configuration
When you first create the widget, you can pass an optional configuration object to set it up. For example, passing in the theme will create the widget with that theme. If this is not passed the configuration will be set by default.
Configuration will persist after the widget is unmounted. You can always update a widget's configuration later by calling the update()
method.
Property | Description |
---|---|
SwapWidgetConfiguration | The configuration type to be used with the Swap Widget. |
- React
- JavaScript
import { checkout } from '@imtbl/sdk';
// When creating the widget, pass in the configuration
// @ts-ignore
const widget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE, {
config: {
language: 'en',
theme: checkout.WidgetTheme.DARK,
// swap flow configuration options
SWAP: {},
},
});
// Update the widget config by calling update()
// @ts-ignore
widget.update({
config: {
theme: checkout.WidgetTheme.LIGHT,
SWAP: {},
},
});
import { checkout } from '@imtbl/sdk';
// When creating the widget, pass in the configuration
const widget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE, {
config: {
language: 'en',
theme: checkout.WidgetTheme.DARK,
// swap flow configuration options
SWAP: {},
},
});
// Update the widget config by calling update()
widget.update({
config: {
theme: checkout.WidgetTheme.LIGHT,
SWAP: {},
},
});
For more information on the configurations across all the Commerce Widgets (e.g. theme) review the Configuration section in our Setup page.
Events
The Commerce Widget emit events events when critical actions have been taken by the user or key states have been reached.
Below is a table outlining the key events associated with a SWAP
flow.
Event Type | Description | Event Payload |
---|---|---|
CommerceEventType.CLOSE | The user clicked the close button on the widget. This should usually be wired up to call the widget's unmount() function. | |
CommerceEventType.SUCCESS | The user has completed the flow successfully. | CommerceSuccessEvent |
CommerceEventType.FAILURE | There has been an error in the flow. | CommerceFailureEvent |
- React
- JavaScript
import { checkout } from '@imtbl/sdk';
//@ts-ignore
const widget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE, {
config: { theme: checkout.WidgetTheme.DARK },
});
// Add event listeners for the SWAP flow
widget.addListener(
checkout.CommerceEventType.SUCCESS,
(payload: checkout.CommerceSuccessEvent) => {
// narrow the event to a successfull swap event
if (payload.type === checkout.CommerceSuccessEventType.SWAP_SUCCESS) {
const { transactionHash } = payload.data;
console.log('successfull swap', transactionHash);
}
}
);
widget.addListener(
checkout.CommerceEventType.FAILURE,
(payload: checkout.CommerceFailureEvent) => {
// narrow the event to a failed swap event
if (payload.type === checkout.CommerceFailureEventType.SWAP_FAILED) {
const { reason, timestamp } = payload.data;
console.log('swap failed', reason, timestamp);
}
}
);
widget.addListener(checkout.CommerceEventType.CLOSE, () => {
widget.unmount();
console.log('widget closed');
});
// Remove event listeners for the SWAP flow
widget.removeListener(checkout.CommerceEventType.SUCCESS);
widget.removeListener(checkout.CommerceEventType.FAILURE);
widget.removeListener(checkout.CommerceEventType.CLOSE);
// Add event listeners for the SWAP flow
widget.addListener(ImmutableCheckout.CommerceEventType.SUCCESS, (payload) => {
// narrow the event to a successfull swap event
if (
payload.type === ImmutableCheckout.CommerceSuccessEventType.SWAP_SUCCESS
) {
const { transactionHash } = payload.data;
console.log('successfull swap', transactionHash);
}
});
widget.addListener(ImmutableCheckout.CommerceEventType.FAILURE, (payload) => {
// narrow the event to a failed swap event
if (payload.type === ImmutableCheckout.CommerceFailureEventType.SWAP_FAILED) {
const { reason, timestamp } = payload.data;
console.log('swap failed', reason, timestamp);
}
});
widget.addListener(ImmutableCheckout.CommerceEventType.CLOSE, () => {
widget.unmount();
console.log('widget closed');
});
// Remove event listeners for the SWAP flow
widget.removeListener(ImmutableCheckout.CommerceEventType.SUCCESS);
widget.removeListener(ImmutableCheckout.CommerceEventType.FAILURE);
widget.removeListener(ImmutableCheckout.CommerceEventType.CLOSE);
Sample code
This sample code gives you a good starting point for integrating the SWAP flow into your application and listening to its events.
- React
- JavaScript
import { useEffect, useState } from 'react';
import { checkout } from '@imtbl/sdk';
// create Checkout SDK
const checkoutSDK = new checkout.Checkout();
export function App() {
const [widget, setWidget] =
useState<checkout.Widget<typeof checkout.WidgetType.IMMUTABLE_COMMERCE>>();
// Initialise widget and mount a SWAP flow
useEffect(() => {
(async () => {
const factory = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK, language: 'en' },
});
const checkoutWidget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE);
setWidget(checkoutWidget);
})();
}, []);
// mount widget and add event listeners
useEffect(() => {
if (!widget) return;
// Swap 100 USDC worth of IMX
widget.mount('mount-point', {
flow: checkout.CommerceFlowType.SWAP,
amount: '100',
direction: checkout.SwapDirection.TO,
fromTokenAddress: 'native', // IMX
toTokenAddress: '0x3B2d8A1931736Fc321C24864BceEe981B11c3c57', // USDC
});
widget.addListener(
checkout.CommerceEventType.SUCCESS,
(payload: checkout.CommerceSuccessEvent) => {
const { type, data } = payload;
// detect successful swap
if (type === checkout.CommerceSuccessEventType.SWAP_SUCCESS) {
console.log('successfull swap', data.transactionHash);
}
}
);
widget.addListener(
checkout.CommerceEventType.FAILURE,
(payload: checkout.CommerceFailureEvent) => {
const { type, data } = payload;
// detect when user fails to swap, bridge, or on-ramp tokens
if (type === checkout.CommerceFailureEventType.SWAP_FAILED) {
console.log('failed to swap', data.reason);
}
}
);
// remove widget from view when closed
widget.addListener(checkout.CommerceEventType.CLOSE, () => {
widget.unmount();
});
// clean up event listeners
return () => {
widget.removeListener(checkout.CommerceEventType.SUCCESS);
widget.removeListener(checkout.CommerceEventType.CLOSE);
widget.removeListener(checkout.CommerceEventType.FAILURE);
};
}, [widget]);
return <div id="mount-point" />;
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/checkout-sdk/dist/browser/index.js"></script>
</head>
<body>
<div id="mount-point"></div>
<script>
// Initialize Checkout SDK
var checkout;
var wrappedBrowserProvider;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const factory = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK, language: 'en' },
});
const widget = factory.create(
ImmutableCheckout.WidgetType.IMMUTABLE_COMMERCE,
{
config: {
WALLET: { showNetworkMenu: false },
},
}
);
// Swap 100 USDC worth of IMX
widget.mount('mount-point', {
flow: checkout.CommerceFlowType.SWAP,
amount: '100',
direction: checkout.SwapDirection.TO,
fromTokenAddress: 'native', // IMX
toTokenAddress: '0x3B2d8A1931736Fc321C24864BceEe981B11c3c57', // USDC
});
widget.addListener(
ImmutableCheckout.CommerceEventType.SUCCESS,
(payload) => {
const { type, data } = payload;
// detect successful swap
if (
type === ImmutableCheckout.CommerceSuccessEventType.SWAP_SUCCESS
) {
console.log('successfull swap', data.transactionHash);
}
}
);
widget.addListener(
ImmutableCheckout.CommerceEventType.FAILURE,
(payload) => {
const { type, data } = payload;
// detect failed swap
if (
type === ImmutableCheckout.CommerceFailureEventType.SWAP_FAILED
) {
console.log('failed to swap', data.reason);
}
}
);
// remove widget from dom when closed
widget.addListener(ImmutableCheckout.CommerceEventType.CLOSE, () => {
widget.unmount();
});
// clean up event listeners
window.addEventListener('beforeunload', () => {
widget.removeListener(ImmutableCheckout.CommerceEventType.SUCCESS);
widget.removeListener(ImmutableCheckout.CommerceEventType.FAILURE);
widget.removeListener(ImmutableCheckout.CommerceEventType.CLOSE);
});
})();
</script>
</body>
</html>